Naming Conventions
Avoid Using Bad Names - A good variable name allows the code to be used by many developers. The name should reflect what it does.
Bad:

Good:

Avoid Hungarian Notation - The Hungarian Notation restates the type which is already present in the declaration. The modern IDEs will identify the type.
Bad:

Good:

The Hungarian Notation should not be used in parameters too.
Bad:

Good:

Avoid Misleading Names - Name the variables to reflect what it is used for.
Bad:

Good:

Use Consistent Capitalization - Do use PascalCasing for all public member, type, and namespace names consisting of multiple words. Do use camel casing for parameter names.
Bad:

Good:

Use Pronounceable Names - It takes time to investigate the meaning of the variables and functions when they are not pronounceable.
Bad:

Good:
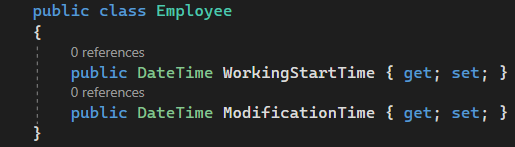
Use Camelcase Notation - Use Camelcase notation for method parameters and variables.
Bad:

Good:

Variables
Too many if-else statements can make the code hard to follow. Explicit is better than implicit.
Bad:

Good:

Avoid Mental Mapping - Don’t force the reader of your code to translate what the variable means.
Bad:

Good:
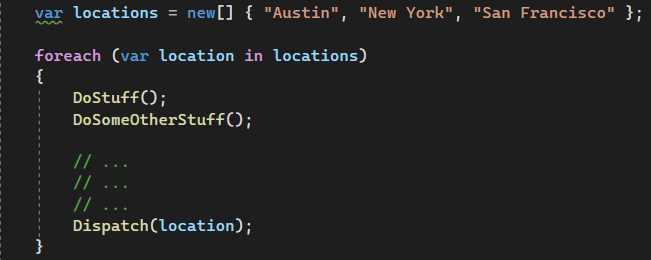
Avoid Magic String - Magic strings are string values that are specified directly within the application code that have an impact on the application’s behavior. Such strings will end up being duplicated within the system, and since they cannot automatically be updated using refactoring tools, they become a common source of bugs when changes are made to some strings but not others.
Bad:
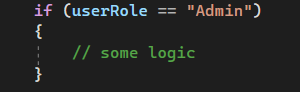
Good:

Don't Add Unneeded Context - If your class/object name tells you something, don't repeat that in your variable name.
Bad:

Good:
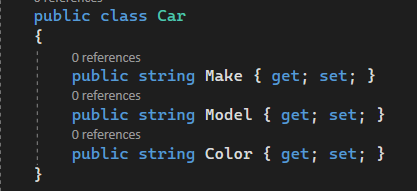
Use Meaningful and Pronounceable Variable Names
Bad:

Good:

Use The Same Vocabulary for the Same Type of Variable
Bad:
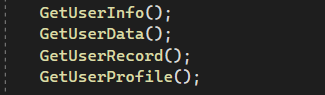
Good:
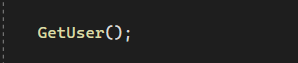
Use Searchable Names - We read more code than we ever write. It's important that the code we do write is readable and searchable. By not naming variables that end up being meaningful for understanding our program, we hurt our readers. Make your names searchable.
Bad:

Good:
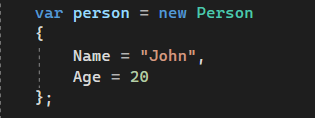
Bad:

Good:

Functions
Avoid Negative Conditionals
Bad:

Good:

Avoid Type-Checking
Bad:
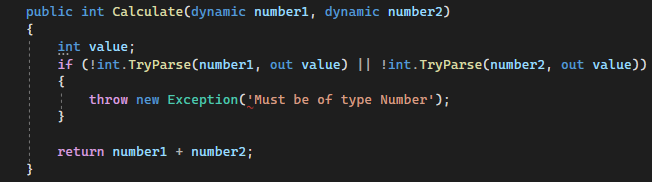
Good:

Avoid Flags In Method Parameters - A flag indicates that the method has more than one responsibility. It is very best if the method only has a single responsibility. So, split the method into two if a boolean parameter adds multiple responsibilities to the method.
Bad:

Good:

Function Arguments (2 or fewer ideally) - Limiting the number of function parameters is incredibly important because it makes testing your function easier.
Bad:

Good:

Classes
Function Names Should Say What They Do
Bad:
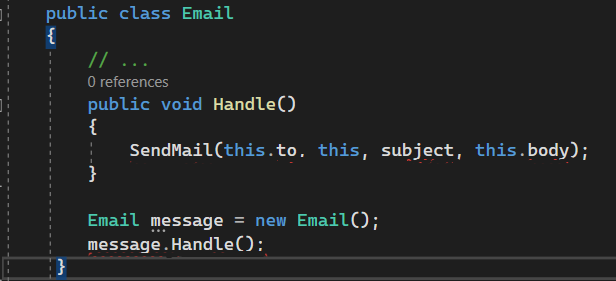
Good:

Remove Dead Code
Bad:
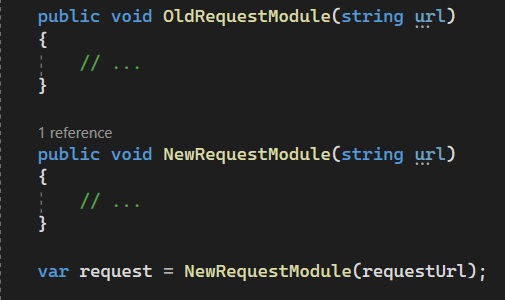
Good:

Use Method Chaining - This pattern is very useful and commonly used in many libraries. It allows your code to be expressive. Use method chaining and look at how clean your code will be.
Good:

Error Handling
Don't Use 'throw ex' In Catch Block - If you need to re-throw an exception after catching it, use just 'throw'. By using this, you will save the stack trace. But in the bad option below, you will lose the stack trace.
Bad:

Good:

Don't Ignore Caught Errors - If you wrap any bit of code in a try/catch it means you think an error may occur there and therefore you should have a plan, or create a code path, for when it occurs.
Bad:

Good:

Use Multiple Catch Blocks Instead of If Conditions - If you need to take action according to the type of exception, you better use multiple catch blocks for exception handling.
Bad:

Good:

Comments
Don't Leave Commented Out Code in Your Codebase
Bad:

Good:

Pay attention to Formatting
Formatting your code improves code readability. Use Tabs over Spaces.
Bad:

Good:
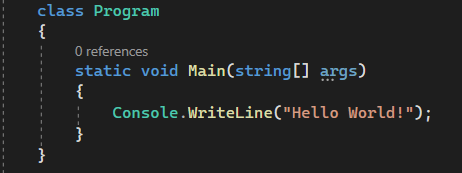
Add Comments Whenever Necessary - This is something we developers really don't like to do.
However, adding a few lines of comments/descriptions of what the method actually does helps you and the other developers. VS makes it very easy for you. Simply Go above any method and type in ///.
VS automatically generates a comment template for you including the arguments of the method.
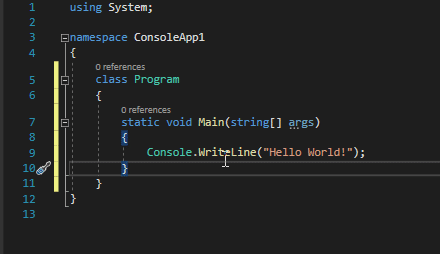
Whenever you call this method (from anywhere), VS will show your comment too. Add comments when a particular method is too complex and requires an in-depth explanation.
Use Async/Await
Asynchronous Programming helps improve overall efficiency while dealing with functions that can take some time to finish computing. While such a function is being executed, the complete application may seem to be frozen to the end-user. This results in a bad user experience. In such cases, we use async methods to free the main thread.
The Proper Way to Do a Null-Check - We perform null-checks quite often in our code, to guard against the dreaded NullReferenceException.
Bad:

The == operator can be overridden and there is no strict guarantee that comparing an object with null will produce the expected result.
There is a new operator that we can use, the is operator.
Good:

Introducing Using Declarations
Using statements in the past always assumed an additional level of nesting in our code that was not necessary:

with C# 8, we can remove the curly braces from the using statements in our code:

Remove If-Else Statements For Setting Boolean Values
We often encounter a situation in our code where we need to return a bool value from a function:
Bad:

Good:
We can simplify the method by simply returning the value of that logical expression.

Good:
We can further simplify the previous method by using an expression body:

Use Ternary Operator
Bad:

Good:

The Proper Way to Return Empty Collections - Often, we have methods that return a collection. We perform some validation beforehand, then populate the collection and return it. However, what should we return in case the preconditions are not met?
Bad:

Good:
A better approach would be to just return an empty collection:
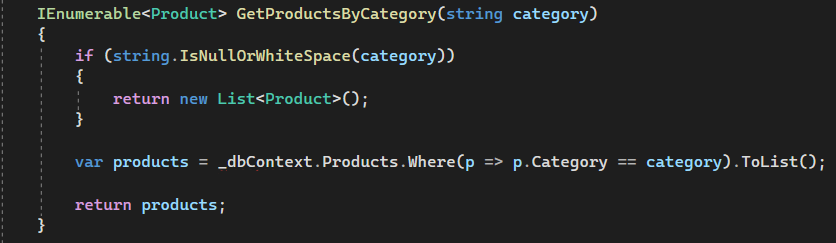
Good:
We can achieve the same result with a cleaner approach:

Use Null Coalescing Operator
Here is a GetProduct function that takes in a Product object as a parameter and checks for the null object.
If null, return a new Object with data, else return the same object.
Bad:

Good:

Prefer String Interpolation - Starting with C# 6, the String Interpolation feature was introduced. This provides a more readable and cool syntax to create formatted strings. Here is how you use interpolated strings.
Bad:

Good:

Use Expression Bodied Methods - Use an Expression body where the method body is much smaller than even the method definition itself. Don’t waste Brackets and lines of code.
Bad:

Good:

How to Simplify Switch Statements - Switch statements can be very useful when we want to evaluate some object, and based on the set of possible values return a different result.
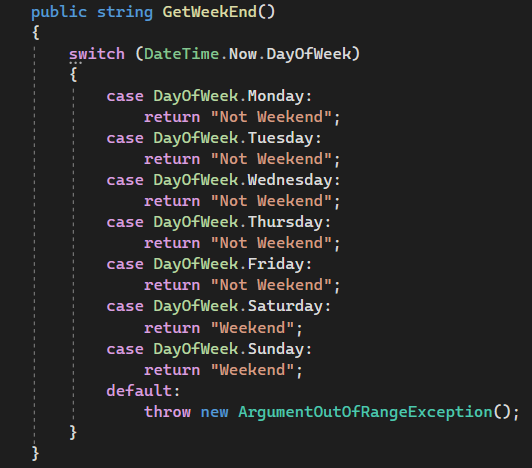
We can compact this further by joining all of the case statements that return the same result.

We can do even better than this. Beginning with C# 8, switch expressions were introduced to help us reduce the amount of code we have to write even more. We can transform the previous switch statement into a switch expression.
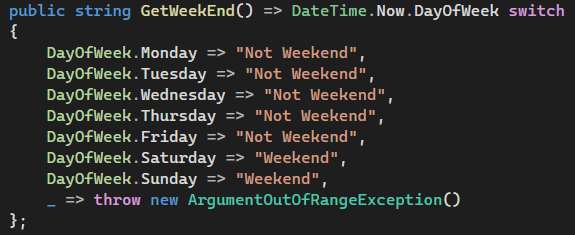
One last optimization we can introduce is to use the negation logical pattern.

Enable Code Clean Up on Save
Starting in Visual Studio 2022 17.1 Preview 2, developers can now perform Code Cleanup automatically when a file is being saved. Code Cleanup automatically on Save is a new feature integrated into Visual Studio 2022 that can clean up your code file to make sure it is formatted correctly and that your coding style preferences are applied. Some customizable preferences include Format documents, Sort usings, Removing unnecessary usings, and more.
This feature can help minimize stylistic violations within PRs and allow developers to spend less time fixing code not meeting specific standards and more time doing what they do best. To give it code cleanup on save a try.

How to enable Code Cleanup on Save
Go to the Analyze >> Code Cleanup >> Configure Code Cleanup to personalize desirable customizations to your code cleanup profile(s).

Configure the fixers you would like to apply on save.
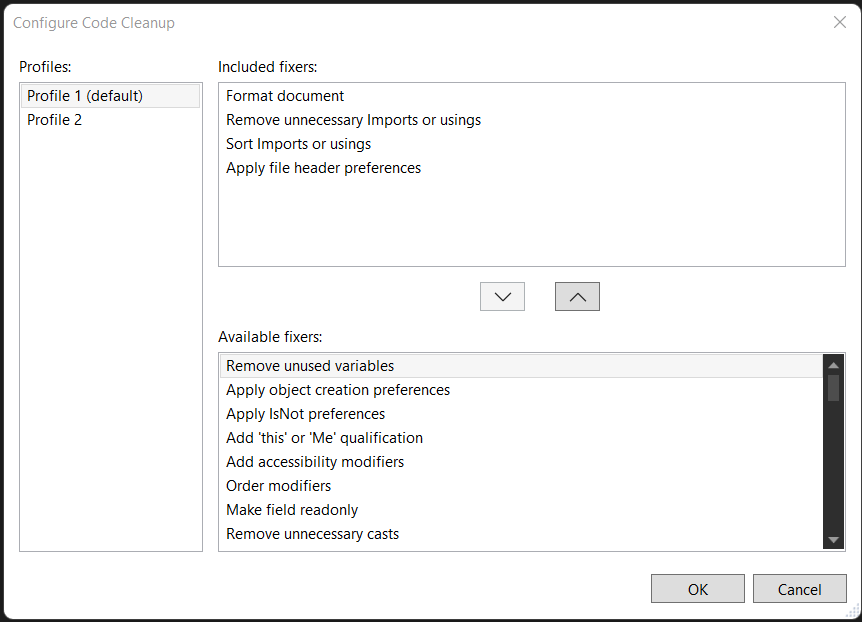
Now Go to Tools >> Options ?> Text Editor >> Code Cleanup. Add a check in the “Run Code Cleanup profile on Save”. Be sure to select the appropriate profile you want to execute automatically whenever you save.
