Hangfire with ASP.NET Core
All applications need to do some sort of background work. A repetitive job that we need to do every day or every other day or weekly or monthly. In this article we are going to learn how we can easily implement it using Hangfire.
What Is Hangfire??
-
Hangfire is an open source library to schedule and execute background jobs in .NET applications. It’s simple to use, well structured, and gives a powerful performance.We can create a simple background process inside the same application pool or thread without creating separate applications.
When To Use Background Processing??
-
Processing a task in the background means that we can execute it away from the application’s main thread.To keep our applications running smoothly and our users happy, for certain tasks we can utilize a concept called background processing. E.g. we may need to send a notification to our user. We can do that behind the scenes and the user can continue to browse freely.
Hangfire Features
-
Easy to install
-
Easy to configure
-
Nice UI dashboard to check up on our jobs at any time
-
Open source software and is completely free
-
Supports multiple queue processing
-
Background jobs are created in a persistent storage – SQL Server and Redis
-
Self-maintainable (We don't need to perform manual storage clean-up)
-
If a job fails, Hangfire will try to run it again as soon as possible.
How does it work?
There are three main components of Hangfire architecture.
Client:
-
The actual libraries inside our application.
-
The client creates the job, serializes its definition, and makes sure to store it into our storage (e.g SQL database).
Server:
-
The server picks up the job definitions from the storage and executes them.
-
The server can live within our application or can be on another server because it always points to the database storage.
-
It also keeps our job storage clean of any data that we don’t use anymore.
Storage:
-
This is our database.
-
It uses some tables that Hangfire default creates for us.
-
It stores all the information about our jobs definitions and execution status.
-
By default, it uses SQL Server, but it also supports RDBMS and NoSQL.
Flow execution
-
First we need to specify the task in the code.
-
Then call the appropriate hangfire method to create it.
-
Based on that the hangfire client creates the job and stores it into the database.
-
Then hangfire returns the control back to the application so the next code can continue with its execution.
-
The server picks up the job stored in the storage and creates a background thread to process the job.

Project setup
Program.cs file
-
Now let’s configure the hangfire in the program.cs file.
-
Add AddHangfire() method with the SQL Server storage.
-
Add the Hangfire server with the AddHangfireServer().
-
Then update our request processing pipeline.
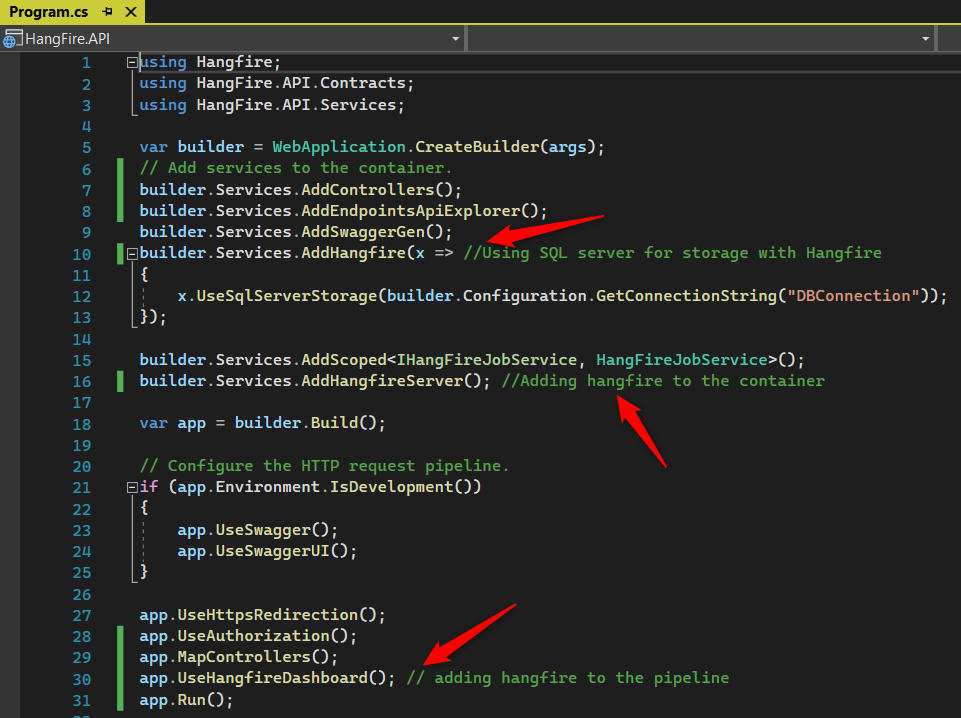

-
Once the application runs successfully, navigate to the https://localhost:7079/hangfire/ hangfire URL.
-
Create an interface IHangFireJobService.cs and Create some methods in it to understand hangfire jobs.
-
Create a HangFireJobService.cs file to implement our interface.
Types of Jobs
-
Fire-And-Forgot Jobs
-
Delayed Jobs
-
Recurring Jobs
-
Continuations Jobs
-
Batches Jobs
-
Batch Continuations Jobs
Now let’s look at each job in detail.
Fire - And - Forgot Jobs

-
Create a [HttpGet("/FireAndForgetJob")] endpoint into the controller.
-
In the controller, we are using the IBackgroundJobClient interface of hangfire.
-
Here we call the interface’s Enqueue() method of IBackgroundJobClient and pass it our FireAndForgetJobAsync() service method below.
-
The Enqueue method will create and save job definitions to our storage.
-
Send the Get request from Swagger.
-
This will create our first job with Hangfire.
-
Now check the job in the dashboard.
-
Go to the Jobs tab and observe our job is available in the Succeeded section.

Delayed Job

-
Create a [HttpGet("/DelayedJob")] endpoint into the controller.
-
In the controller, we are again using the IBackgroundJobClient interface of hangfire.
-
Here we call the interface’s Schedule() method instead of Enqueue() method of the IBackgroundJobClient hangfire interface and pass it our DelayedJobAsync() service method.
-
Here we added the delay.
-
The Schedule method will also create the job definition and save it
-
It will also schedule it in the queue at the specified time.
-
Send the Get request from Swagger.
-
We scheduled a job with a delay of 60 seconds.
-
Go to the Job>> Scheduled section.

Recurring Job

-
Create a [HttpGet("/RecurringJob")] endpoint into the controller.
-
In the controller,we are using the IRecurringJobManager interface of hangfire.
-
Here we call the interface’s AddOrUpdate() method of IRecurringJobManager interface and pass it our RecurringJobAsync() service method.
-
We also need to pass the job id and the CRON interval.
-
The AddOrUpdate method will create a new job for the first time.
-
Now check the job in the dashboard.
-
Go to the Recurring tab and observe our job is available.

Continuations Jobs

-
Create a [HttpGet("/ContinuationJob")] endpoint into the controller.
-
In the controller, we are again using the IBackgroundJobClient interface of hangfire.
-
First we will create one Fire and Forget job then
-
This job will trigger the next job, so we will get the id from the Enqueue() method once it’s created.
-
Then call the interface ContinueJobWithAsync() method and pass the job id of the created job and call the ContinueJobWithAsync() method
-
The ContinueJobWithAsync() method will create a chain for our two jobs together.

Output of all the jobs executed

Real World Example using hangfire
-
Now,let's implement one real world scenario using a Recurring job to send an email.
-
Write some method to add a simple GET method to send an email using smtp client.
-
Call it by using AddOrUpdate() method of IRecurringJobManager interface.

Note: Not adding here the sample code to send email using SMTP!
Reference: https://www.hangfire.io/
Enjoy coding!!!