class B { //B is not allowed to extend any other class other than object
method(){
....
}
}
class A with B {
....
......
}
void main() {
A a = A();
a.method(); //we got the method without inheriting B
}
The code class A with B
is equivalent to class A extends Object with B
. Right now, classes can only be used as mixins once they only extend object. Classes which extend other classes cannot be used as a mixin. This could change in the future however since Dart is continuously evolving and there is already an experimental feature which allows us to use mixins which extends class other than Object
. Maybe at the time of reading this article there could be some minor changes how mixins behave. So always keep an eye on mixin specifications.
Although we can’t use mixin class which extends class other than Object
, we can extends or implement the base class(Class A
in this example) which is using mixin class:
class B { //B is not allowed to extend any other class other than object
method(){
....
}
}
class C {
...
...
}
class A extends C with B { . //we can extends of implement class A
....
......
}
void main() {
A a = A();
assert(a is B); // a is b = true
assert(a is C); // a is c = true
a.method(); //we got the method without inheriting B
}
Mixins enables a programming language to mimic an benefit from features such as multiple inheritance and code reuse while also reducing the risk of running into problems such as the Deadly Diamond of Death(DDD).
Some people describe mixins as an interface with implementations.
What is deadly diamond of death(DDD) in multiple inheritance?
Suppose we have an abstract class called Performer.
abstract class Performer {
void perform();
}
Now we will make two concrete classes Dancer and Singer which extends Performer.
class Dancer extends Performer {
void perform() {
print('Dance Dance Dance ');
}
}
class Singer extends Performer {
void perform() {
print('lalaaa..laaalaaa....laaaaa');
}
}
Extending Performer
is the only way I can dance and sing.
Now suppose dart supports multiple inheritance and a class called Musician extends both the classes Dancer and Singer.
Note: Dart only supports Single inheritance, I’m just assuming here, what will happen if dart supported Multiple Inheritance like C++:
class Musician extends Dancer,Singer {
void showTime() {
perform();
}
}
Can you guess which perform()
method will be called in Musician class?
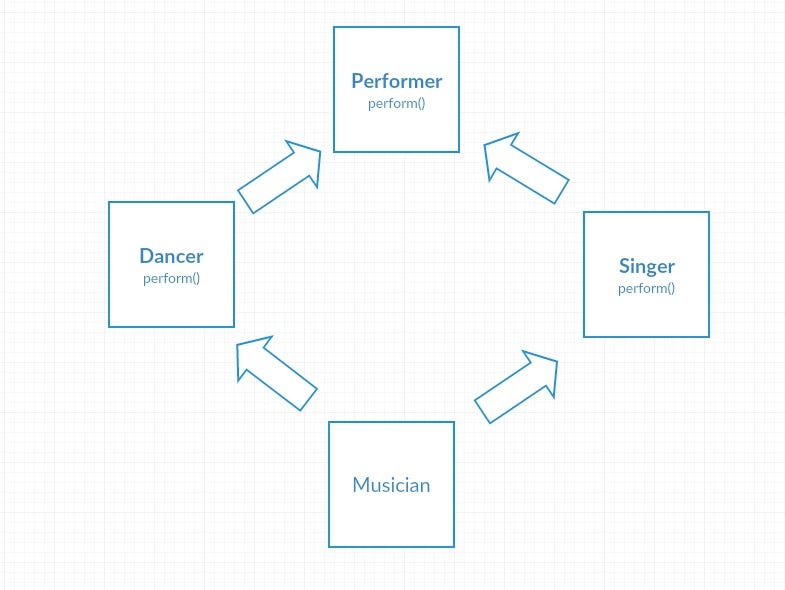
Confused? This is what a compiler feels when we try to run this code.Because there’s no actual way for us to decide which is the right method to call. This very situation is known as deadly diamond of death and it is a core problem of multiple inheritance. Thankfully, we can fix this using mixins.
What mixin can do for us?
As the official docs states:
“To implement a mixin, create a class that extends Object and declares no constructors. Unless you want your mixin to be usable as a regular class, use the mixin
keyword instead of class
.”
The mixin
keyword was introduced in dart 2.1 and it is analogous to abstract class
in some ways and different from it in other way. Like abstract class
, you can declare abstract methods in mixins and it cannot be instantiated. But unlike abstract
, you can’t extend a mixin.
You can either use the keyword mixin
, abstract class
or a normal class as a mixin. Let’s take a look at how we can solve the above problem with mixins.
Always keep in mind that mixin is not multiple inheritance instead they are just a way to reuse our code from a class in multiple hierarchies without needing to extend them. Its like being able to use other people assets without being their children. Its cool hah! 😝
First we have to create our Performer
class with method perform()
,
class Performer {
void perform() {
print('performing...');
}
}
Next we create the class Dancer which contains a method named perform()
mixin Dancer {
void perform() {
print('Dance...Dance...Dance..');
}
}
Now we will declare another class called Singer which will contain the same method perform()
mixin Singer {
void perform() {
print('lalaaa..laaalaaa....laaaaa');
}
}
Lets create one more class Musician which will use both of these classes along with Performe
class:
class Musician extends Performer with Dancer,Singer {
void showTime() {
perform();
}
}
Now if we try to run the above code, we will get the output lalaaa..laaalaaa....laaaa
, can you guess why ?
When mixing classes, the classes used as mixins are not parallel but instead on top of the class using it. This is the reason why methods do not conflict with one another, allowing us to use codes from multiple classes without having to deal with the vulnerabilities of multiple inheritance.
If you are confused by this, don’t panic, I will explain how mixins are evaluated.
For determining which class method will be executed when using mixins, follow these simple steps:
- If the class using mixins is extending any class then put that class on top of the stack. i.e
class Musician extends Performer
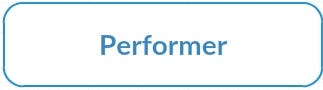
- Always remember the order in which you declare mixins, this is very important because it decides which class is more important. If mixins contains identical methods then the mixin class that is declared later will be executed(Declaring a mixin after another raises its “Importance”).
In our example, we declared Dancer
before the Singer, so it is more “Important”and therefore would go below Performer
. I.e class Musician extends Performer with Dancer
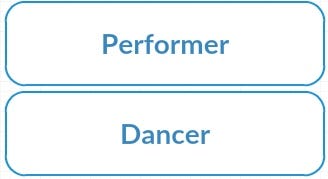
- After
Dancer
we declared Singer
, so put the Singer
below Dancer
in the stack :
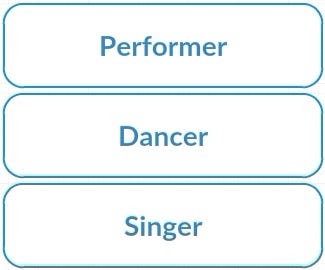
- Finally, add the class which is using mixins i.e
Musician
into the stack. This class would be the most important or most specific class.If this class contains any identical methods to mixins or super class then the methods in this class would be called first…always.
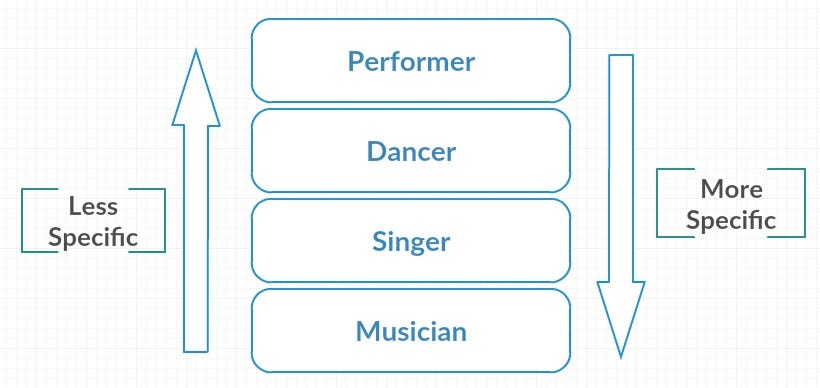
Got it? The idea behind how mixins can be used as a way to reuse code without having to deal with multiple inheritance and the problems which arise because of it.
Now we will try to focus on some other advanced stuffs that we can do with mixins.
Doing some more stuff with mixin!
Although we can do many normal and advanced stuff using Dart’s mixins, there is one more thing which we need to look at and that is the keyword on
. The keyword on
is used to restrict our mixin’s use to only classes which either extends
or implements
the class it is declared on. In order to use the on
keyword, you must declare your mixin using the mixin
keyword.
Below is an example of using the On
keyword:
class A {}
class B{}
mixin X on A{}
mixin Y on B {}
class P extends A with X {} //satisfies condition as it extends A
class Q extends B with Y {} //satisfies condition as it extends B
In the above code we are restricting mixins X
and Y
to be only used by the classes which either implements or extends A
and B
respectively.