Cross-Site Scripting (XSS) attacks are a type of injection, in which malicious scripts are injected into otherwise benign and trusted websites. XSS attacks occur when an attacker uses a web application to send malicious code, generally in the form of a browser side script, to a different end user.
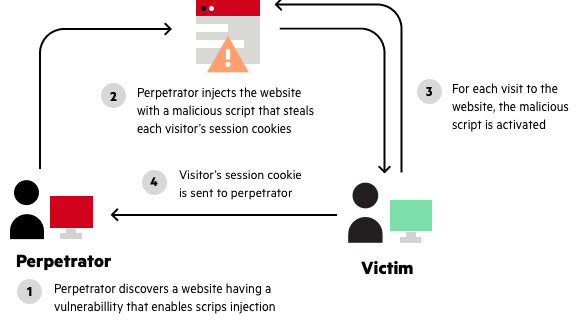
Steps of prevention for your Angular application:
-
Install xss package.
npm install -- save xss
-
Create a function in new file xss-errors.ts
import { AbstractControl, ValidationErrors } from '@angular/forms';
import xss from 'xss';
export function XssError(control: AbstractControl): ValidationErrors | null {
const value: string = control.value;
if (value === undefined || value === null || value.length === 0) {
return undefined;
}
const strResult = xss(value, {
whiteList: {},
stripIgnoreTag: true,
stripIgnoreTagBody: ['script'],
});
if (strResult.includes('[removed]')) {
return { XssError: true };
}
return undefined;
}
-
ng add @angular/material
-
In app.component.html
<form class="form" [formGroup]="formGroup">
<h2>XSS Demo</h2>
<input class="input" formControlName="firstName" />
<br/>
<div class="errorMessage" *ngIf="!!firstName.errors && firstName.errors['required']">Required!</div>
<div class="errorMessage" *ngIf="!!firstName.errors && firstName.errors['XssError']">Invalid name!</div>
<br/>
<a class="button" (click)="save()">Save</a>
</form>
-
In app.component.ts
import { Component, OnInit } from '@angular/core';
import { FormControl, FormGroup, Validators } from '@angular/forms';
import { XssError } from './xss-errors';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss'],
})
export class AppComponent implements OnInit {
title = 'angular-xss';
formGroup: FormGroup;
ngOnInit(): void {
this.formGroup = new FormGroup({
firstName: new FormControl('Anuja', [Validators.required, XssError]),
});
}
save(): void {
if (this.formGroup.invalid) {
return;
}
}
get firstName() { return this.formGroup.get('firstName'); }
}
-
In app.component.scss
.form {
padding: 20px;
}
.errorMessage {
color: red;
font-weight: 700;
padding: 2px;
}
.input {
padding: 10px;
}
.button {
border-radius: 5px;
border: 1px solid white;
background-color: pink;
padding: 15px;
cursor: pointer;
}
Output:



