This article demonstrates how you can use Firebase Authentication to sign in a user by sending an SMS message to the user's phone. The user signs in using a one-time code contained in the SMS message.
Requirements:
1. Android Studio 3.4
2. If you haven't already,add Firebase to your Android project
1.Firebase Register Project Steps:
Step:1.1:
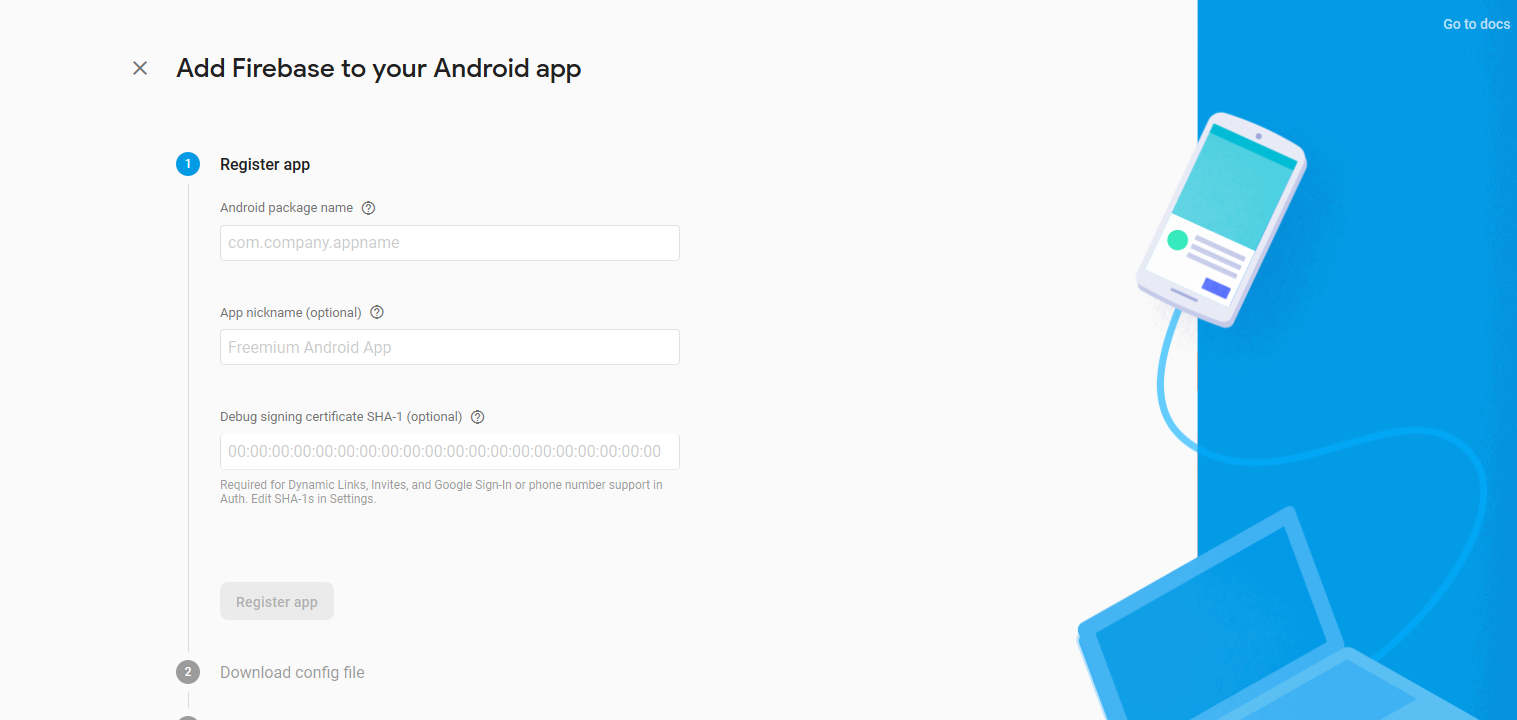
Step:1.2:
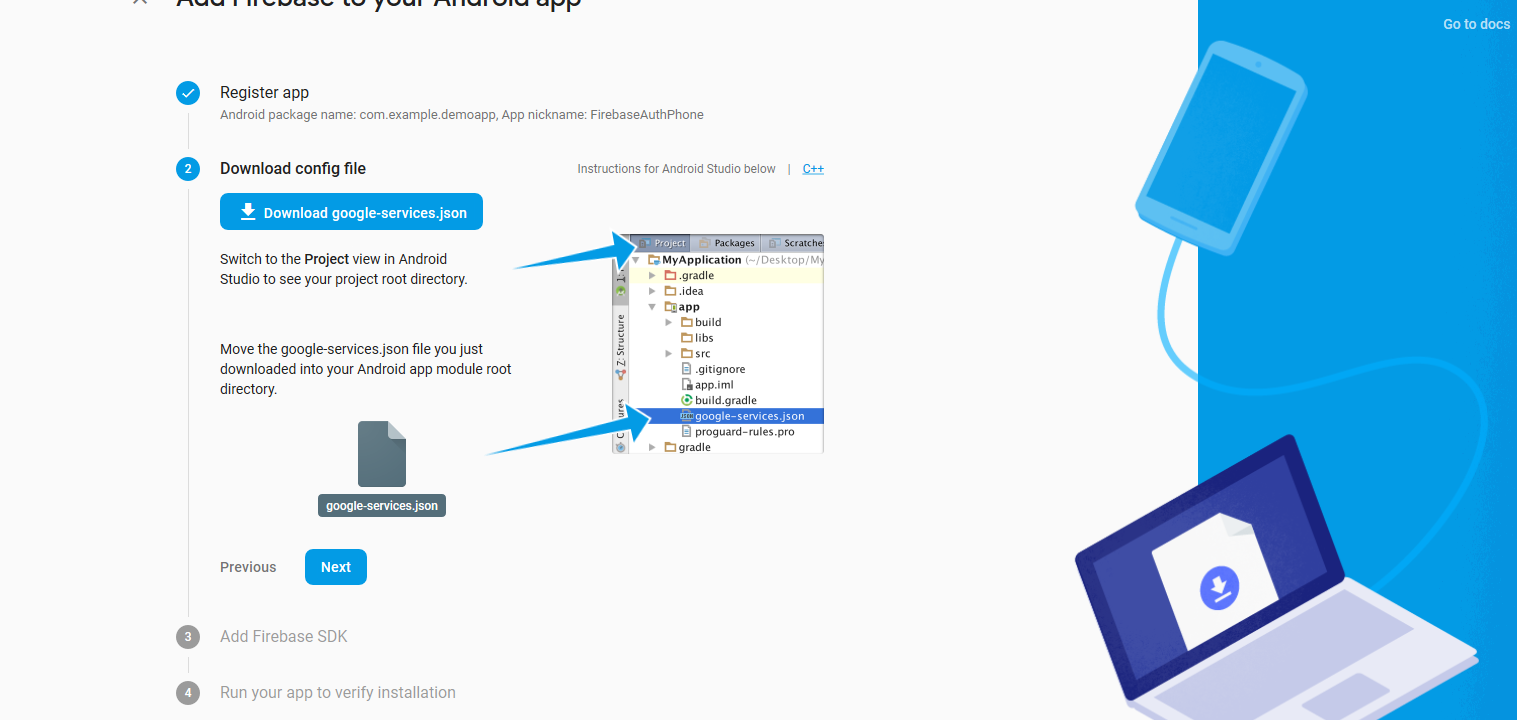
Step:1.3:
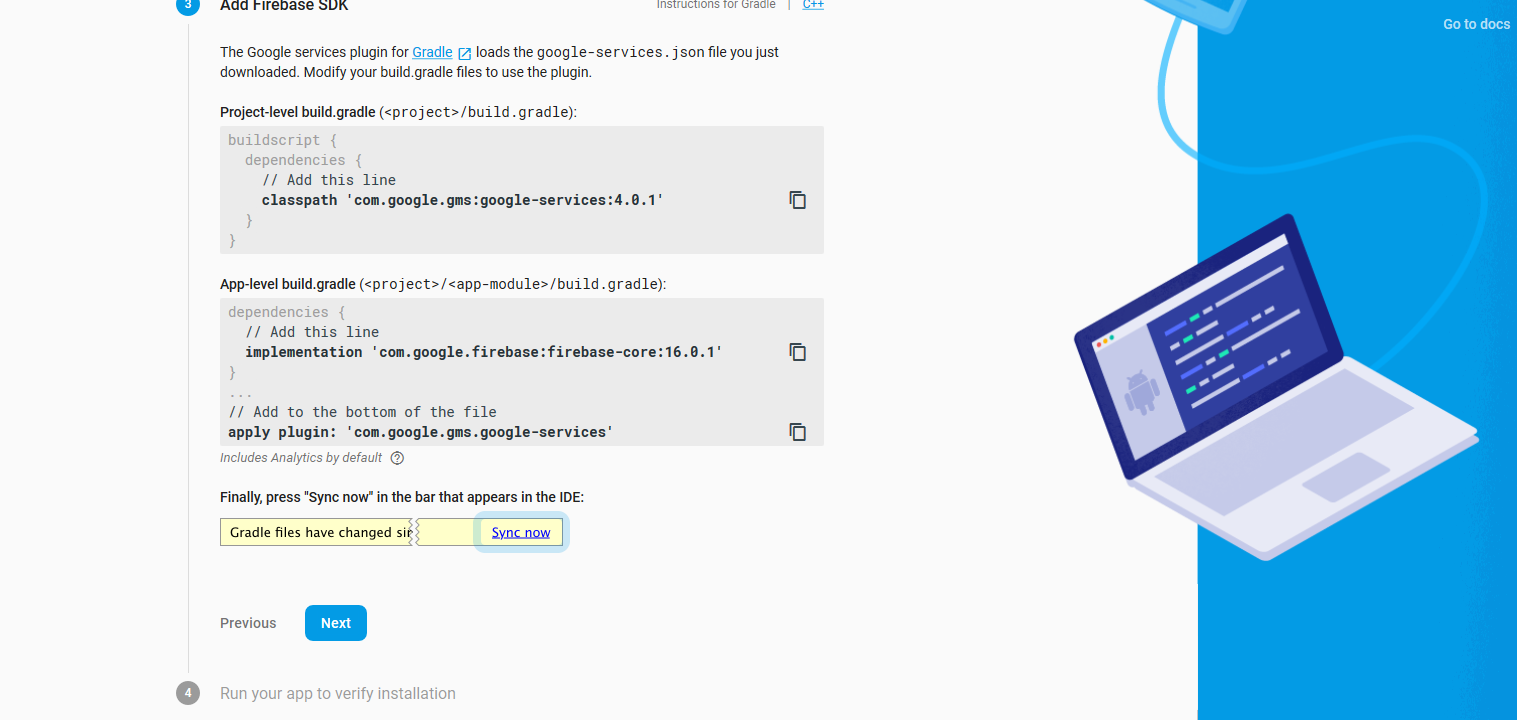
Step:1.4:

2.Once the Firebase setup is complete, go to the Firebase console and follow these steps:
Click on Develop from the left navigation bar and then click Authentication.
Step:2.1:

Step:2.2:Now, navigate to SIGN-IN METHOD tab:


Step:2.3: Enable the Phone Authentication.

Security concerns:
Authentication using only a phone number, while convenient, is less secure than the other available methods, because possession of a phone number can be easily transferred between users. Also, on devices with multiple user profiles, any user that can receive SMS messages can sign in to an account using the device's phone number.
3.Required Layout file
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_margin="8dp"
android:orientation="vertical"
tools:context=".FirebasePhoneNubmerAuthActivity">
<EditText
android:id="@+id/phoneNumberEditText"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="22dp"
android:ems="10"
android:hint="Enter phone number"
android:inputType="phone"
android:maxLength="10"
android:maxLines="1" />
<Button
android:id="@+id/sendOTPButton"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="15dp"
android:text="Send Otp" />
<EditText
android:id="@+id/otpNumberEditText"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="15dp"
android:ems="6"
android:hint="Enter verification OTP"
android:inputType="number"
android:maxLength="6"
android:maxLines="1"
android:visibility="gone" />
<Button
android:id="@+id/verifyOTPButton"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="15dp"
android:text="Verify OTP"
android:visibility="gone" />
</LinearLayout>
4.In the Java file, let's start by implementing basic code:
public class FirebasePhoneNubmerAuthActivity extends AppCompatActivity implements View.OnClickListener{
EditText phoneNumberEditText, otpNumberEditText;
Button sendOTPButton, verifyOTPButton;
private FirebaseAuth mAuth;
PhoneAuthProvider.OnVerificationStateChangedCallbacks mCallbacks;
String mVerificationId;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_firebase_phone);
phoneNumberEditText = (EditText)findViewById(R.id.phoneNumberEditText);
otpNumberEditText = (EditText)findViewById(R.id.otpNumberEditText);
sendOTPButton = (Button)findViewById(R.id.sendOTPButton);
verifyOTPButton = (Button)findViewById(R.id.verifyOTPButton);
sendOTPButton.setOnClickListener(this);
verifyOTPButton.setOnClickListener(this);
mAuth = FirebaseAuth.getInstance();
initFireBaseCallbacks();
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.sendOTPButton:
String mobile = phoneNumberEditText.getText().toString().trim();
if(mobile.isEmpty() || mobile.length() < 10){
phoneNumberEditText.setError("Enter a valid mobile Number");
phoneNumberEditText.requestFocus();
}else {
phoneNumberEditText.setError(null);
PhoneAuthProvider.getInstance().verifyPhoneNumber(
"+91" + mobile,1, TimeUnit.MINUTES,this,mCallbacks);
}
break;
case R.id.verifyOTPButton:
String otpCode = otpNumberEditText.getText().toString().trim();
if (otpCode.isEmpty() || otpCode.length() < 6) {
otpNumberEditText.setError("Enter valid code");
otpNumberEditText.requestFocus();
}else {
PhoneAuthCredential credential = PhoneAuthProvider.getCredential(mVerificationId,otpCode);
mAuth.signInWithCredential(credential)
.addOnCompleteListener(this, new OnCompleteListener() {
@Override
public void onComplete(@NonNull Task task) {
if (task.isSuccessful()) {
FirebaseUser user = task.getResult().getUser();
Toast.makeText(FirebasePhoneNubmerAuthActivity.this, "Verification Success", Toast.LENGTH_SHORT).show();
} else {
if (task.getException() instanceof FirebaseAuthInvalidCredentialsException) {
Toast.makeText(FirebasePhoneNubmerAuthActivity.this, "Verification Failed, Invalid credentials", Toast.LENGTH_SHORT).show();
}
}
}
});
}
break;
}
}
void initFireBaseCallbacks() {
mCallbacks = new PhoneAuthProvider.OnVerificationStateChangedCallbacks() {
@Override
public void onVerificationCompleted(PhoneAuthCredential credential) {
}
@Override
public void onVerificationFailed(FirebaseException e) {
Toast.makeText(FirebasePhoneNubmerAuthActivity.this, "Verification Failed", Toast.LENGTH_SHORT).show();
}
@Override
public void onCodeSent(String verificationId,
PhoneAuthProvider.ForceResendingToken token) {
Toast.makeText(FirebasePhoneNubmerAuthActivity.this, "OTP Sent Successfully", Toast.LENGTH_SHORT).show();
mVerificationId = verificationId;
phoneNumberEditText.setVisibility(View.GONE);
sendOTPButton.setVisibility(View.GONE);
otpNumberEditText.setVisibility(View.VISIBLE);
verifyOTPButton.setVisibility(View.VISIBLE);
}
};
}
}